How to Integrate Firebase Dynamic Links to iOS Apps
How to Integrate Firebase Dynamic Links to iOS Apps
Have you ever wanted to be informed when your app is installed for the first time by a user that is redirected to App Store via a link? This is the main question that led me to the Firebase Dynamic Links
This framework lets you to know when the app is installed via the dynamic link you have created on Firebase or the app is opened by clicking this link which is known as universal linking.
This blog post aims to show you how to create a dynamic link on Firebase console and integrate it properly to your iOS app.
First, click on the link console.firebase.google.com and create a new iOS app and do not forget to add App Store ID and Team ID in the project settings. This is essential to let your dynamic link know which App Store page will be opened when it is interacted on an iPhone where your app is not installed.
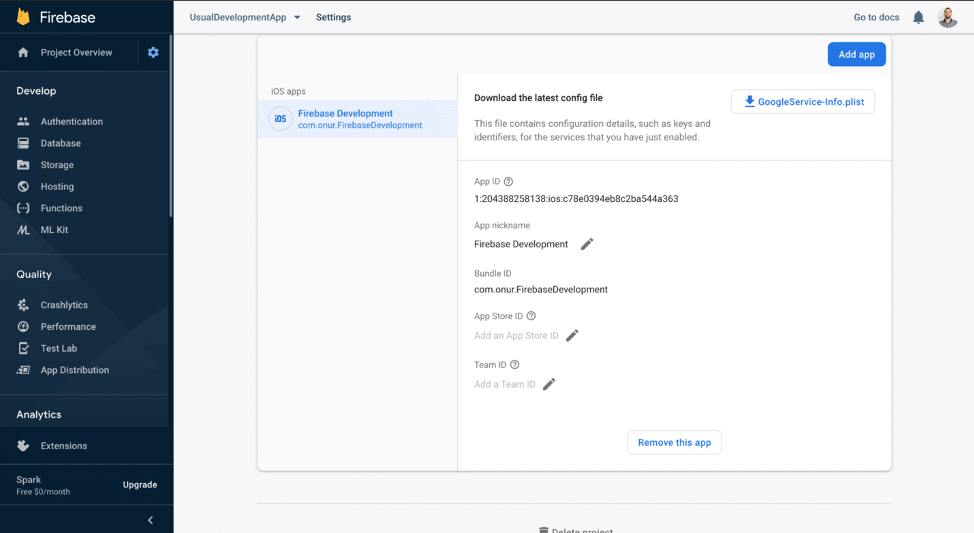
Then, tap on the Dynamic Links in the left menu and create your first dynamic link. You can add an existing domain as dynamic link domain, but you need to verify that the domain belongs to your organization.
How to verify your domain
I also prefer another option which is creating custom dynamic link domain. Firebase creates Apple App Site Association file automatically for custom dynamic link url: usualdevelopmentapp.page.link/apple-app-site-association. The Apple App Site Association is a file that should be in your domain and specified with appID to make universal linking unique.
After setting this domain, tap on New Dynamic Link button to set up dynamic link details properly.
- First step is to set up short link URL. This is the public URL that user interacts to install or launch your app.
- At the second step, you need to set a deep link URL which is handled by your app to initialize it with a custom logic. Deep link must be a valid URL because any user can open short link in a desktop web browser will be redirected to this link.
- At the third step, if the app is not installed to the device, you should determine whether to redirect to the app store or open a custom URL in the web browser.
- Step four is the same link defining behaviour but this time for the android app.
- Step five is an optional step to set a preview page which will be opened when user tapped on the short link that you have created. You can set the image, title and description for the preview page or you can prefer to skip this page and redirect the user directly to the application. Firebase does not recommend to skipping preview page. According to the documentation:
“The app preview page increases your click to install rate. It more reliably sends users to the appropriate destination (app or store) when they click on your link in social apps in a more reliable way.”
When all the configurations are ready on the Firebase console do not forget to enable Associated Domains option for your app in developer.apple.com.
Now, all configurations are done. Open the Xcode and add the dynamic link URL to the associated domains in the Signing & Capabilities section.
iOS can be aware of the dynamic linking in two different ways. The first one is to click the short link on a device that the app is already installed in and then launch the app. This way is also known as Universal Linking. The second one is that, install the app from the app store with the redirection of the link. These two ways are handled in different methods in the AppDelegate file.
func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool { guard userActivity.activityType == NSUserActivityTypeBrowsingWeb, let url = userActivity.webpageURL, let host = url.host else { return false } let isDynamicLinkHandled = DynamicLinks.dynamicLinks().handleUniversalLink(url) { dynamicLink, error in guard error == nil, let dynamicLink = dynamicLink, let urlString = dynamicLink.url?.absoluteString else { return } print("Dynamic link host: \(host)") print("Dyanmic link url: \(urlString)") print("Dynamic link match type: \(dynamicLink.matchType.rawValue)") } return isDynamicLinkHandled }
Handling dynamic links that received as Universal Link
If the user clicks on the short link which you created on the console when the app is installed on the device, this method will be called and it will print:
Dynamic link host: usualdevelopmentapp.page.link Dynamic link url: https://usualdevelopmentapp.page.link/urlParameter?parameter=value Dynamic link match type: 3
Host is the main host of your dynamic link.
URL is the deep link url that is embedded on the dynamic link in the second step above. You can initialize your app with the logic that depending on this URL.
Match type value of dynamic link is unique whose raw value is 3 when the app is already installed on the device and opened by clicking the link.
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any] = [:]) -> Bool { if let dynamicLink = DynamicLinks.dynamicLinks().dynamicLink(fromCustomSchemeURL: url) { guard let urlString = dynamicLink.url?.absoluteString else { return false } print("Dyanmic link url: \(urlString)") print("Dynamic link match type: \(dynamicLink.matchType.rawValue)") return true } return false }
Handling dynamic links that received as Custom URL
When the user clicks on the link on a device where the app is not installed and redirected the app store then installs and launchs the app, this is the method that will be triggered by iOS.
Dynamic link url:https://usualdevelopmentapp.page.link/urlParameter?parameter=value Dynamic link match type: 2
URL is the same as expected but this time match type has changed.
Match type value of dynamic link is default whose raw value is 2 when the app is installed to the device from the app store and launched for the first time via the link.
Throughout the post, I tried to share with you what I have learned from integrating Firebase Dynamic Links to the app that I am working on. I hope this will be helpful for you and feedbacks are really appreciated.
Reading Time: 7 minutes
Don’t miss out the latestCommencis Thoughts and News.
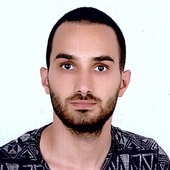
Onur Kara / Software Engineer
05/03/2020
Reading Time: 7 minutes
Have you ever wanted to be informed when your app is installed for the first time by a user that is redirected to App Store via a link? This is the main question that led me to the Firebase Dynamic Links
This framework lets you to know when the app is installed via the dynamic link you have created on Firebase or the app is opened by clicking this link which is known as universal linking.
This blog post aims to show you how to create a dynamic link on Firebase console and integrate it properly to your iOS app.
First, click on the link console.firebase.google.com and create a new iOS app and do not forget to add App Store ID and Team ID in the project settings. This is essential to let your dynamic link know which App Store page will be opened when it is interacted on an iPhone where your app is not installed.
Don’t miss out the latestCommencis Thoughts and News.
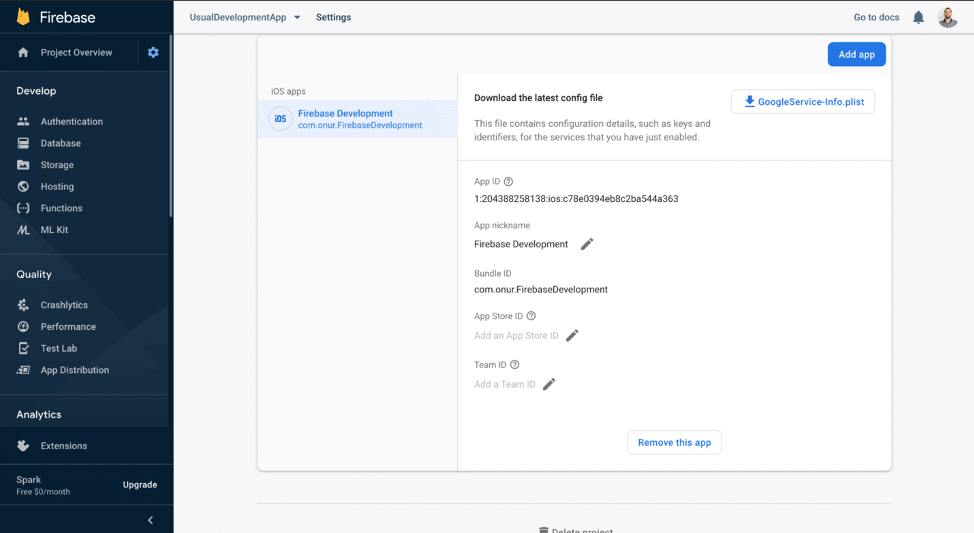
Then, tap on the Dynamic Links in the left menu and create your first dynamic link. You can add an existing domain as dynamic link domain, but you need to verify that the domain belongs to your organization.
How to verify your domain
I also prefer another option which is creating custom dynamic link domain. Firebase creates Apple App Site Association file automatically for custom dynamic link url: usualdevelopmentapp.page.link/apple-app-site-association. The Apple App Site Association is a file that should be in your domain and specified with appID to make universal linking unique.
After setting this domain, tap on New Dynamic Link button to set up dynamic link details properly.
- First step is to set up short link URL. This is the public URL that user interacts to install or launch your app.
- At the second step, you need to set a deep link URL which is handled by your app to initialize it with a custom logic. Deep link must be a valid URL because any user can open short link in a desktop web browser will be redirected to this link.
- At the third step, if the app is not installed to the device, you should determine whether to redirect to the app store or open a custom URL in the web browser.
- Step four is the same link defining behaviour but this time for the android app.
- Step five is an optional step to set a preview page which will be opened when user tapped on the short link that you have created. You can set the image, title and description for the preview page or you can prefer to skip this page and redirect the user directly to the application. Firebase does not recommend to skipping preview page. According to the documentation:
“The app preview page increases your click to install rate. It more reliably sends users to the appropriate destination (app or store) when they click on your link in social apps in a more reliable way.”
When all the configurations are ready on the Firebase console do not forget to enable Associated Domains option for your app in developer.apple.com.
Now, all configurations are done. Open the Xcode and add the dynamic link URL to the associated domains in the Signing & Capabilities section.
iOS can be aware of the dynamic linking in two different ways. The first one is to click the short link on a device that the app is already installed in and then launch the app. This way is also known as Universal Linking. The second one is that, install the app from the app store with the redirection of the link. These two ways are handled in different methods in the AppDelegate file.
func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool { guard userActivity.activityType == NSUserActivityTypeBrowsingWeb, let url = userActivity.webpageURL, let host = url.host else { return false } let isDynamicLinkHandled = DynamicLinks.dynamicLinks().handleUniversalLink(url) { dynamicLink, error in guard error == nil, let dynamicLink = dynamicLink, let urlString = dynamicLink.url?.absoluteString else { return } print("Dynamic link host: \(host)") print("Dyanmic link url: \(urlString)") print("Dynamic link match type: \(dynamicLink.matchType.rawValue)") } return isDynamicLinkHandled }
Handling dynamic links that received as Universal Link
If the user clicks on the short link which you created on the console when the app is installed on the device, this method will be called and it will print:
Dynamic link host: usualdevelopmentapp.page.link Dynamic link url: https://usualdevelopmentapp.page.link/urlParameter?parameter=value Dynamic link match type: 3
Host is the main host of your dynamic link.
URL is the deep link url that is embedded on the dynamic link in the second step above. You can initialize your app with the logic that depending on this URL.
Match type value of dynamic link is unique whose raw value is 3 when the app is already installed on the device and opened by clicking the link.
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any] = [:]) -> Bool { if let dynamicLink = DynamicLinks.dynamicLinks().dynamicLink(fromCustomSchemeURL: url) { guard let urlString = dynamicLink.url?.absoluteString else { return false } print("Dyanmic link url: \(urlString)") print("Dynamic link match type: \(dynamicLink.matchType.rawValue)") return true } return false }
Handling dynamic links that received as Custom URL
When the user clicks on the link on a device where the app is not installed and redirected the app store then installs and launchs the app, this is the method that will be triggered by iOS.
Dynamic link url:https://usualdevelopmentapp.page.link/urlParameter?parameter=value Dynamic link match type: 2
URL is the same as expected but this time match type has changed.
Match type value of dynamic link is default whose raw value is 2 when the app is installed to the device from the app store and launched for the first time via the link.
Throughout the post, I tried to share with you what I have learned from integrating Firebase Dynamic Links to the app that I am working on. I hope this will be helpful for you and feedbacks are really appreciated.
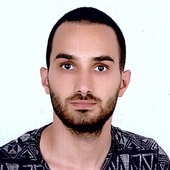